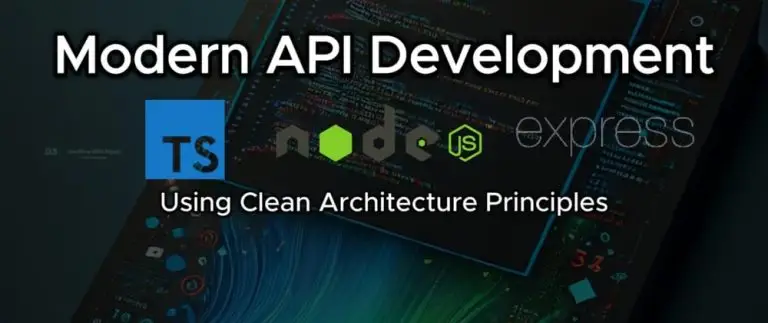
APIs are the backbone of modern web applications. As the complexity of applications grows, it’s crucial to adopt an architecture that promotes scalability, maintainability, and testability. In this blog, we’ll explore how to build a modern API using Node.js, Express, and TypeScript, all while adhering to Clean Architecture principles.
1. 🧩 Introduction to Clean Architecture
Clean Architecture, introduced by Robert C. Martin (Uncle Bob), emphasizes the separation of concerns within an application. It promotes the idea that the business logic should be independent of any frameworks, databases, or external systems. This makes the application more modular, easier to test, and adaptable to changes.
Key principles of Clean Architecture:
- Independence: The core business logic should not depend on external libraries, UI, databases, or frameworks.
- Testability: The application should be easy to test without relying on external systems.
- Flexibility: It should be easy to change or replace parts of the application without affecting others.
2. 💡 Why Node.js, Express, and TypeScript?
Node.js
Node.js is a powerful JavaScript runtime that allows you to build scalable network applications. It’s non-blocking and event-driven, making it ideal for building APIs that handle a large number of requests.
Express
Express is a minimalistic web framework for Node.js. It provides a robust set of features for building web and mobile applications and APIs. Its simplicity makes it easy to start with, and it’s highly extensible.
TypeScript
TypeScript is a superset of JavaScript that adds static types. Using TypeScript in your Node.js application helps catch errors early in the development process, improves code readability, and enhances the overall developer experience.
3. 🚧 Setting Up the Project
First, let’s create a new Node.js project and set up TypeScript.
mkdir clean-architecture-api
cd clean-architecture-api
npm init -y
npm install express
npm install typescript @types/node @types/express ts-node-dev --save-dev
npx tsc --init
Next, configure your tsconfig.json
:
{
"compilerOptions": {
"target": "ES2020",
"module": "commonjs",
"strict": true,
"esModuleInterop": true,
"skipLibCheck": true,
"forceConsistentCasingInFileNames": true,
"outDir": "./dist"
},
"include": ["src/**/*.ts"],
"exclude": ["node_modules"]
}